Table of contents
- Introduction
- Environment Setup
- Create Project and Install dependencies
- Basic Screens
- StackNavigator
- BottomTabNavigator
- Conclusion
Introduction
In a web browser, you can link to different pages using an anchor (<a>
) tag. When the user clicks on a link, the URL is pushed to the browser history stack. When the user presses the back button, the browser pops the item from the top of the history stack, so the active page is now the previously visited page. React Native doesn’t have a built-in idea of a global history stack like a web browser does — this is where React Navigation enters the story.
React Navigation’s stack navigator provides a way for your app to transition between screens and manage navigation history. If your app uses only one stack navigator then it is conceptually similar to how a web browser handles navigation state — your app pushes and pops items from the navigation stack as users interact with it, and this results in the user seeing different screens.
Environment Setup
Following should be installed on your system:
- Npm or Yarn
- Node.js
- Latest
expo-cli
Create Project and Install dependencies
Create a new Expo project with a blank
template by running the following command in a terminal window and install dependencies required for react-navigation:
expo init sampleProject -- npm # npm flag for using npm as package manager, defualt is yarn
cd sampleProject
expo install @react-navigation/native @react-navigation/bottom-tabs @react-navigation/stack ereact-native-gesture-handler react-native-reanimated react-native-screens react-native-safe-area-context @expo/vector-icons @react-native-community/masked-view
Basic Screens
In the root project folder, create a new directory screens
and inside it, create three new files called Home.js, Detail.js
and Setting.js
import React from 'react'
import { StyleSheet, View, Text, Button } from 'react-native'
function Home({ navigation }) {
return (
<View style={styles.container}>
<Button
onPress={()=>navigation.navigate('Detail')}
title="Go to Detail Screen"
color="#841584"
/>
</View>
)
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#ffffff'
},
})
export default Home
Same code can be used for other screens.
StackNavigator
As we have already mentioned about stack navigation in introduction, let’s try it with a practical example. Create a new folder navigation, inside it create a file named StackNavigator.js
and add the following code:
import * as React from 'react'
import { createStackNavigator } from '@react-navigation/stack'
import Home from '../screens/Home'
import Home from '../screens/Detail'
import Home from '../screens/Setting'
const Stack = createStackNavigator() function StackNavigator(){
return (
<Stack.Navigator>
<Stack.Screen name='Home' component={Home} />
<Stack.Screen name='Detail' component={Detail} />
<Stack.Screen name='Setting' component={Setting} />
</Stack.Navigator>
)
}
export default StackNavigator
The createStackNavigator
is a function used to implement a stack navigation pattern. This function returns two React components: Screen
and Navigator
, which help us configure each component screen.
In the above snippet, there are two required props with each Stack.Screen
. The prop name
refers to the name of the route, and the prop component
specifies which screen to render at the particular route.
Don’t forget to export the StackNavigator
since it’s going to be imported in the root of the app, (that is, inside App.js
file) as shown below:
import React from 'react' import MainStackNavigator from './src/navigation/MainStackNavigator' import { NavigationContainer } from '@react-navigation/native'
export default function App() {
return (
<NavigationContainer>
<BottomTabNavigator />
</NavigationContainer>
)
}
The NavigationContainer
is a component that manages the navigation tree. It also contains the navigation state and has to wrap all the navigator’s structure. Execute the command expo start
and make sure the Expo client is running either in a simulator device or a real device. You should see the Home screen, like this:
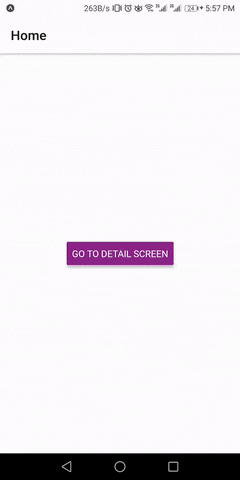
BottomTabNavigator
Now, lets take a look at BottomTabNavigator and How can we configure it. Create a file named BottomTabNavigator.js
and add the following code:
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
import * as React from 'react';
import { MaterialIcons } from '@expo/vector-icons';
function TabBarIcon(props) {
return (
<MaterialIcons
name={props.name}
size={30}
style={{ marginBottom: -3 }}
color={props.focused ? '#841584' : '#ccc'}
/>
);
}
import Home from '../screens/Home'
import Detail from '../screens/Detail'
import Setting from '../screens/Setting'
const BottomTab = createBottomTabNavigator();
const INITIAL_ROUTE_NAME = 'Home';
export default function BottomTabNavigator({ navigation, route }) {
return (
<BottomTab.Navigator initialRouteName={INITIAL_ROUTE_NAME}
tabBarOptions={{ activeTintColor: '#841584' }}
>
<BottomTab.Screen
name="Home"
component={Home}
options={{
title: 'Home',
tabBarIcon: ({ focused }) => <TabBarIcon focused={focused} name="home" />,
}}
/>
<BottomTab.Screen
name="Detail"
component={Detail}
options={{
tabBarLabel: 'Detail',
tabBarIcon: ({ focused }) => <TabBarIcon focused={focused} name="details" />,
}}
/>
<BottomTab.Screen
name="Settings"
component={Setting}
options={{
title: 'Setting',
tabBarIcon: ({ focused }) => <TabBarIcon focused={focused} name="settings" />,
}}
/>
</BottomTab.Navigator>
);
}
Change the App.js file with following code:
import React from 'react';
import BottomTabNavigator from './navigation/BottomTabNavigator'
import { NavigationContainer } from '@react-navigation/native'
export default function App() {
return (
<NavigationContainer>
<BottomTabNavigator />
</NavigationContainer>
)
}
Now, you should see the Home screen, like this:
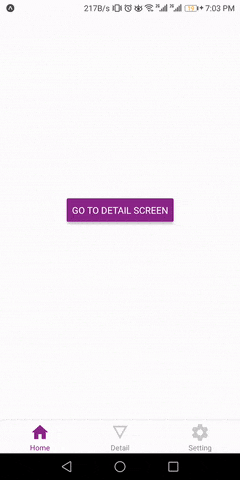
Conclusion
In this article, we have implemented StackNavigator and BottomTabNavigator in react-navigation V5. There are also other Navigator’s and we will cover them in our next post. I hope you liked this article, please feel free to ask anything related in the comment section, Thanks!
References Official Documentation link.